Simple Commission Calculation Program Part 2 Explanation
Simple Commission Calculation Program Part 2 - Answered by a verified Programmer. PRG 420 Week 2 Individual Assignment Simple Commission Calculation Program Part 1. Write a Java™ application using NetBeans™ Integrated Development Environment.
Details
PRG 420 Week 2 Simple Commission Calculation Program Part 1
Write a Java™ application using NetBeans™ Integrated Development Environment (IDE) that calculates the total annual compensation of a salesperson. Either a GUI graphic user interface) program or non-GUI program is acceptable. Consider the following factors:
• A salesperson will earn a fixed annual salary of $25,000.00.
• A salesperson will also receive a commission as a sales incentive. Commission is a percentage of the salesperson’s annual sales. The current commission is 10% of total sales.
• The total annual compensation is the fixed salary plus the commission earned.
The Java™ application should meet these technical requirements:
Create a NetBeans project and name it XXXIA2. XXX is your last name. It is important to use your last name so that each student’s project name is unique. When your team evaluation the members’ program, the team will know whom the program belong to. Your system can also load in multiple projects without conflicting project names. The number 2 in the file name is the academic week number.
• The application should have at least one class, in addition to the application’s controlling class (a controlling class is where the main function resides).
• There should be proper documentation in the source code.
• The application should ask the user to enter annual sales, and it should display the total annual compensation.
Because NetBeans produces multiple files for one project, the best method to submit an assignment is to zip the files. There should be one project folder created by NetBeans using your project name. Zip the folder. Submit your NetBeans project zip file.
Sorry, but copying text is forbidden on this website!
PRG420 (Version 10) – Week 4 Individual- Simple Commission Calculation Program Part 3
Modify the Week Three Java™ application using Java™ NetBeans™ IDE to meet these additional and changed business requirements:
* The application will now compare the total annual compensation of at least two salespersons. * It will calculate the additional amount of sales that each salesperson must achieve to match or exceed the higher of the two earners. * The application should ask for the name of each salesperson being compared.
The Java™ application should also meet these technical requirements:
* The application should have at least one class, in addition to the application’s controlling class.
Amv codec serial numbers. Amv Codec Serial. UpdateStar is compatible with Windows platforms. UpdateStar has been tested to meet all of the technical requirements to be compatible with.
We will write a custom essay on Prg420 V10 Week 4 Individual Assignment specifically for you
for only$16.38$13.90/page
* The source code must demonstrate the use of Array or ArrayList. * There should be proper documentation in the source code.
Source Code:
/**
*
Program: Simple Commission Calculation Program Part 3
Purpose: to calculates and display the total annual compensation of a salesperson. Programmer:
Class: PRG420
Instructor:
Creation Date:
Programmer Modification Date:
Purpose: to add name of sales person and also functionality to manage the list of sales persons an comparing their annual compensation Program Summary: This program will calculate and display the total annual compensation of a salesperson. Here in this program the salary of the salesman and its commission rate is fixe and program accepts sales amount.
*/
import java.util.ArrayList;
import java.util.Scanner;
import java.text.NumberFormat;
Simple Commission Calculation Program Part 2
class SalesPerson {
private final double fixed_Salary = 35750.00;
private final double commission_Rate = 12.0;
private final double sales_Target = 125250.00;
private String name;
private double annual_Sales;
//default constructor
public SalesPerson() {
name = “Unknown”;
annual_Sales = 0.0;
}
//parameterized constructor
public SalesPerson(String nm,double aSale) {
name = nm;
annual_Sales = aSale;
}
//getter method for the name
public String getName(){
return name;
}
Gharshana Audio Information: Cast And Crew: Venkatesh, Asin Director: Gautham Menon Producer: C.Venkat Raju, G.Siva Raju Music: Harris Jayaraj Album Bit Rate: 320/128kbps Language: Telugu Single Download Link Cheliya Cheliya Song – Singer: K.K., Suchitra Nanne Nanne Song – Singer: Tippu, Shalini Singh Andagaada Song – Singer: Harini Aadatanama Song – Singer: Sunitha Sarathy, Fabi Mani Ye Chilipi Song – Singer: Srinivas The Encounter Song – Singer: Harris Jayaraj Zip Download Link 320 Kbps OR 128 Kbps Zip. Download gharshana telugu background music.
//setter method to set name
public void setName(String nm){
name = nm;
}
//getter method for the annual sales
public double getAnnualSales(){
return annual_Sales;
}
//method to set the value of annual sale
public void setAnnualSales(double aSale) {
annual_Sales = aSale;
}
//method to calcualte and get commission
public double commission (){
double commission = 0;
if(annual_Sales>= (sales_Target*(80/100))) {//80% of the sales target
if(annual_Sales>= sales_Target){
commission = sales_Target * (commission_Rate/100.0) + (annual_Sales- sales_Target)* (75.0/100.0); }
else
commission = annual_Sales * (commission_Rate/100.0); }
return commission ;
}
//method to calcualte and get annual compensation
public double annualCompensation (){
return fixed_Salary + commission();
}
}
public class Main {
public static void main(String args[]){
//array list to have a collection of sales persons
ArrayList<SalesPerson> sales_Persons = new ArrayList<SalesPerson>();
//create an object of Scanner calss to get the keyboard input Scanner input = new Scanner(System.in);
do{
//prompt the user to enter name
System.out.print(“Enter salesperson name (stop to EXIT) : “); String name = input.nextLine().trim();
if(name.equalsIgnoreCase(“stop”))
break;
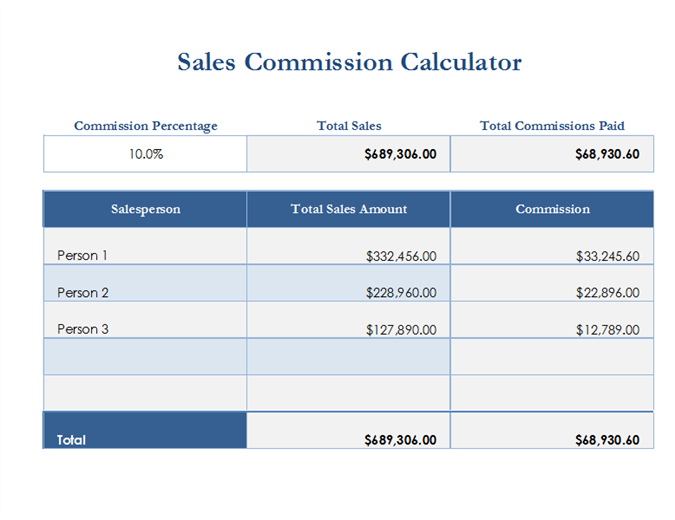
//creating an object of SalesPerson class
SalesPerson sales_Person = new SalesPerson();
//set name of sales person
sales_Person.setName(name);
//prompt the user to enter the annual sales
System.out.print(“Enter the annual sales : “);
double sale = input.nextDouble();
Aquasoft stages 10. //set the value of annual sale of sales person object sales_Person.setAnnualSales(sale);
//add sales Person to array list
sales_Persons.add(sales_Person);
//read a blank line
input.nextLine();
}
while(true);
//getting the 2 minimum annual compensation
double min = -1;
double secondMin = -1;
if(sales_Persons.size()>=3){
//intilization
double firstValue = sales_Persons.get(0).annualCompensation(); double secondValue = sales_Persons.get(1).annualCompensation();

//intechanging if in reverse oreder
if (firstValue < secondValue) {
min = firstValue;
secondMin = secondValue;
}
else {
min = secondValue;
secondMin = firstValue;
}
double nextElement = -1;
//compring the 2 to n values
for (int i = 2; i < sales_Persons.size(); i++) { nextElement = sales_Persons.get(i).annualCompensation(); if (nextElement < min) {
secondMin = min;
min = nextElement;
}
else if (nextElement < secondMin) {
secondMin = nextElement;
}
}
}
//displaying result
NumberFormat nf = NumberFormat.getCurrencyInstance();
//All salespersons and their total annual compensation System.out.println(“n”);
System.out.printf(String.format(“%-20s%-20s”,”Name”, “Total annual compensation” )); System.out.println();
for(SalesPerson salesperson :sales_Persons){
System.out.printf(String.format(“%-20s%20s”,salesperson.getName(), nf.format(salesperson.annualCompensation()))); System.out.println();
}
//dipslyaing the all sales persons additional amount of sales other the 2 memebrs who have minimum sales System.out.println(“n”);
for(int i=0; i< sales_Persons.size();i++){
double compensation = sales_Persons.get(i).annualCompensation();
if(compensation min compensation secondMin) continue;
System.out.println(“Name of Salesperson : “+sales_Persons.get(i).getName()); System.out.println(“The total annual compensation : “+nf.format(compensation));
System.out.println(“Total SalestTotal Compensation”);
double sale = sales_Persons.get(i).getAnnualSales(); for(double j =sale; j<= (sale+ sale * 0.5); j+= 5000.0 ) { sales_Persons.get(i).setAnnualSales(j);
System.out.println(nf.format(j)+”t”+nf.format(sales_Persons.get(i).annualCom
pensation())); }
System.out.println();
}